This article demonstrates how to Create a User Card Component in React.
This code consists of two React components: UserCard.js
and App.js
, showcasing a simple example of a user card rendered in a React application. Let’s break down each part:
UserCard.js
import React from 'react';
const UserCard = (props) => {
return (
<div>
<h2>User Card</h2>
<p>Name: {props.name}</p>
<p>Email: {props.email}</p>
<p>Username: {props.username}</p>
</div>
);
};
export default UserCard;
In the UserCard
component, a functional component is defined. It takes props
as a parameter, which are used to pass data from the parent component (App.js
). Inside the component, a simple HTML structure is rendered, displaying the user’s name, email, and username.
App.js
// App.js
import React, { useState } from 'react';
import UserCard from './UserCard';
function App() {
return (
<div className="App">
<UserCard name="Amit" email="amit@gmail.com" username="amit0001" />
</div>
);
}
export default App;
Output

In the App
component, the UserCard
component is imported. Within the return
statement, a div
with the className “App” is rendered, and an instance of the UserCard
component is used. The name
, email
, and username
props are passed with specific values (“Amit,” “amit@gmail.com,” and “amit0001,” respectively) to customize the displayed information in the user card.
This example illustrates the basic structure of a React application with a parent (App.js
) rendering a child component (UserCard.js
) and passing data between them using props. The UserCard
component can be reused with different user data in various parts of the application.
Further Reading
How to Create Class Components in React?
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
PHP Projects for Undergraduate Students
Architectural Constraints of REST API
Creating a Classified Ads Application in PHP
How to Create a Bar Chart in ReactJS?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
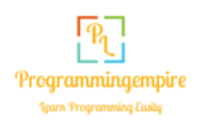