This article demonstrates how to develop a A Product List Component in React JS.
This code comprises two React components: ProductList.js
and App.js
, showcasing the creation of a product list and its integration within a larger React application. Let’s delve into the details of each part:
ProductList.js
import React from 'react';
const ProductList = (props) => {
return (
<div>
<h2>Product List</h2>
<ul>
{props.products.map((product) => (
<li key={product.id}>
{product.name} - ${product.price}
</li>
))}
</ul>
</div>
);
};
export default ProductList;
In the ProductList
component, a functional component is defined that takes a products
prop. Inside the component, a simple HTML structure is rendered, including a heading “Product List” and an unordered list (ul
). The list items (li
) are dynamically generated based on the array of products received as a prop. Each product’s name and price are displayed within list items.
App.js
// App.js
import React, { useState } from 'react';
import UserCard from './UserCard';
import ProductList from './ProductList';
import ColorPicker from './ColorPicker';
import CounterApp from './CounterApp';
import RandomQuoteGenerator from './RandomQuoteGenerator';
import FormValidationApp from './FormValidationApp';
import CounterApp1 from './CounterApp1';
import TimerApp from './TimerApp';
import ToggleVisibilityApp from './ToggleVisibilityApp';
function App() {
const products = [
{ id: 'P001', name: 'Pen', price: 20 },
{ id: 'P002', name: 'Notebook', price: 30 },
{ id: 'P003', name: 'Bag', price: 250 },
];
const [selectedColor, setSelectedColor] = useState('#00ff00'); // Default color is green
// Handler function to update the selected color
const handleColorChange = (newColor) => {
setSelectedColor(newColor);
};
return (
<div className="App">
<UserCard name="Amit" email="amit@gmail.com" username="amit0001" />
<ProductList products={products} />
<ColorPicker selectedColor={selectedColor} onColorChange={handleColorChange} />
<CounterApp />
<RandomQuoteGenerator />
<FormValidationApp />
<CounterApp1 />
<TimerApp />
<ToggleVisibilityApp />
</div>
);
}
export default App;
Output

In the App
component, various React components are imported, including ProductList
. An array of products
is defined, and the ProductList
component is rendered within the main application, passing the products
array as a prop. This demonstrates how to utilize the ProductList
component within a larger React application, showcasing a list of products with their names and prices. Additionally, the code includes the use of other components, such as UserCard
, ColorPicker
, CounterApp
, RandomQuoteGenerator
, FormValidationApp
, CounterApp1
, TimerApp
, and ToggleVisibilityApp
, forming a comprehensive React application.
Further Reading
How to Create Class Components in React?
Examples of Array Functions in PHP
Exploring PHP Arrays: Tips and Tricks
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
PHP Projects for Undergraduate Students
Architectural Constraints of REST API
Creating a Classified Ads Application in PHP
How to Create a Bar Chart in ReactJS?
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
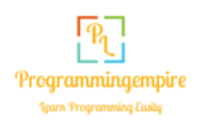