This article demonstrates A Basic Example of Jumbotron Using Bootstrap 4.
Below is a simple HTML file that includes the Bootstrap 4 CDN and has a basic structure with a navigation bar, a jumbotron, and a few content sections:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<title>Bootstrap 4 Basic Structure</title>
</head>
<body>
<!-- Navigation Bar -->
<nav class="navbar navbar-expand-lg navbar-dark bg-dark">
<a class="navbar-brand" href="#">My Website</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item active">
<a class="nav-link" href="#">Home <span class="sr-only">(current)</span></a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Services</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Contact</a>
</li>
</ul>
</div>
</nav>
<!-- Jumbotron -->
<div class="jumbotron">
<div class="container">
<h1 class="display-4">Welcome to My Website!</h1>
<p class="lead">This is a simple example of a website built with Bootstrap 4.</p>
</div>
</div>
<!-- Content Sections -->
<div class="container">
<div class="row">
<div class="col-md-6">
<h2>Section 1</h2>
<p>This is the first content section.</p>
</div>
<div class="col-md-6">
<h2>Section 2</h2>
<p>This is the second content section.</p>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.9.3/dist/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
</body>
</html>
This example includes a responsive navigation bar, a jumbotron for a hero section, and two content sections using the Bootstrap grid system.
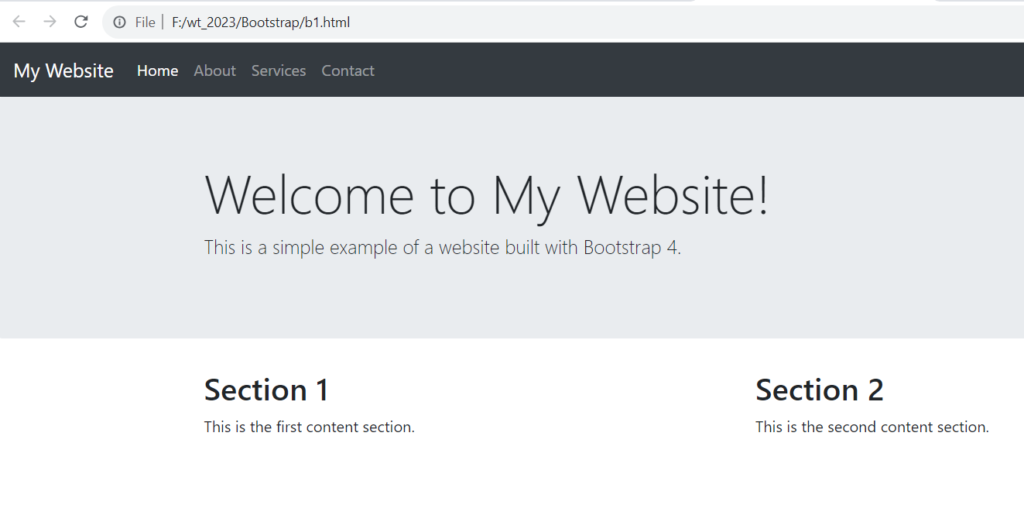
Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Understanding Document Object Model (DOM) in JavaScript
Creating Classes in JavaScript
Bootstrap Frequently Asked Questions
Most Popular Bootstrap Interview Questions and Answers
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
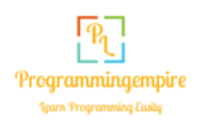