The following code shows an example of using static variable in a function in PHP.
When we use static variable in a function in PHP, it retains its value across function calls. In other words, the value updated by the function for a static variable is retained even after the function returns. Subsequent calls to the same function use the updated values.
The Example Code to Demonstrate Static Variable in a Function in PHP
In the following code, the variable x is static in the function myfunction(). When the first function call updates the value of x to 10, the variable x retains its value. In all subsequent function calls, the update value is used.
<?php
function myfunction()
{
static $x=0;
$y=0;
$x+=10;
$y+=10;
echo 'Value of static variable x = ',$x,'<br>';
echo 'Value of non-static variable y = ',$y,'<br>';
}
echo 'First Function Call...<br>';
myfunction();
echo 'Second Function Call...<br>';
myfunction();
echo 'Third Function Call...<br>';
myfunction();
?>
Output

Apart from using static in a function, we can have static properties and methods in a class also. An example of using static keyword with properties and methods of a class is given here.
Further Reading
Examples of Array Functions in PHP
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
PHP Projects for Undergraduate Students
Architectural Constraints of REST API
Creating a Classified Ads Application in PHP
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
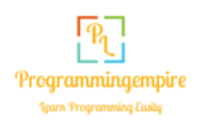