The following program demonstrates How to Perform Thread Synchronization and Inter-thread Communication in Java.
Thread synchronization and inter-thread communication are essential concepts in multithreading to ensure that multiple threads can work together effectively without conflicts or data corruption. Here, I’ll provide you with two Java programs to demonstrate these concepts: one for thread synchronization using synchronized
blocks, and another for inter-thread communication using wait()
and notify()
.
Thread Synchronization Example (Using synchronized
)
In this example, we’ll create two threads that increment a shared counter. To prevent data corruption, we’ll use synchronization to ensure that only one thread can modify the counter at a time.
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
public class SynchronizationDemo {
public static void main(String[] args) {
Counter counter = new Counter();
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 10000; i++) {
counter.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 10000; i++) {
counter.increment();
}
});
thread1.start();
thread2.start();
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Final Count: " + counter.getCount());
}
}
In this program, the Counter
class uses the synchronized
keyword to ensure that only one thread can execute the increment
and getCount
methods at a time. This prevents data corruption and race conditions.
Inter-thread Communication Example (Using wait()
and notify()
)
In this example, we’ll create two threads: one that produces data (Producer) and another that consumes data (Consumer). We’ll use wait()
and notify()
to coordinate their actions.
class SharedResource {
private int data;
private boolean dataProduced = false;
public synchronized void produce(int value) {
while (dataProduced) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
data = value;
dataProduced = true;
System.out.println("Produced: " + value);
notify();
}
public synchronized int consume() {
while (!dataProduced) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
dataProduced = false;
System.out.println("Consumed: " + data);
notify();
return data;
}
}
public class InterThreadCommunicationDemo {
public static void main(String[] args) {
SharedResource resource = new SharedResource();
Thread producerThread = new Thread(() -> {
for (int i = 1; i <= 5; i++) {
resource.produce(i);
}
});
Thread consumerThread = new Thread(() -> {
for (int i = 1; i <= 5; i++) {
int data = resource.consume();
}
});
producerThread.start();
consumerThread.start();
}
}
In this program, the SharedResource
class has methods produce
and consume
, which use wait()
and notify()
to synchronize access to the shared data. The producer thread produces data, and the consumer thread consumes it in a coordinated manner.
These examples demonstrate how to use thread synchronization and inter-thread communication in Java to ensure thread safety and effective communication between threads.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
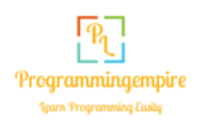