The following code example demonstrates How to Implement User-Defined Exceptions in Java.
Problem Statement
Develop an application in Java to create a user-defined exception class using the extends keyword.
Solution
In order to create a user-defined exception class in Java, you can extend the built-in Exception
class or one of its subclasses. The following code shows an example of how to create and use a custom exception class using the extends
keyword.
// Define a custom exception class by extending Exception
class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
// Create a class to demonstrate the custom exception
public class CustomExceptionDemo {
public static void main(String[] args) {
try {
int result = divide(10, 0);
System.out.println("Result: " + result);
} catch (CustomException e) {
System.out.println("Custom Exception caught: " + e.getMessage());
}
}
public static int divide(int dividend, int divisor) throws CustomException {
if (divisor == 0) {
// Throw the custom exception when divisor is zero
throw new CustomException("Division by zero is not allowed.");
}
return dividend / divisor;
}
}
In this example:
- We define a custom exception class called
CustomException
that extends the built-inException
class. This custom exception class has a constructor that accepts a message as an argument. - In the
CustomExceptionDemo
class, we have adivide
method that throws theCustomException
when the divisor is zero. - In the
main
method, we attempt to divide by zero, which triggers the custom exception. So, we catch and handle this exception in thecatch
block, printing the custom error message.
This demonstrates how to create a user-defined exception class using the extends
keyword and use it to handle specific exceptions in your Java application.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
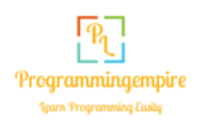