The following example code demonstrates How to Create and Use a Delegate in C#.
Basically, a delegate is a class member that refers to a method. In other words, we can call a method using a delegate. However, the same delegate object can also point to another method with the matching signature.
using System;
namespace DelegatesDemo
{
class Program
{
public int FindLength(string s)
{
return s.Length;
}
static void Main(string[] args)
{
Program ob = new Program();
Func<string, int> ob1 = new Func<string, int>(ob.FindLength);
int n = ob1("Hello, World!!!");
Console.WriteLine("Length = " + n);
}
}
}
Output

Further Reading
How to Create Instance Variables and Class Variables in Python
Comparing Rows of Two Tables with ADO.NET
Example of Label and Textbox Control in ASP.NET
One Dimensional and Two Dimensuonal Indexers in C#
Private and Static Constructors in C#
- Angular
- ASP.NET
- Bootstrap
- C
- C#
- C++
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
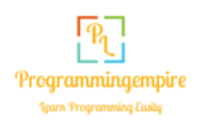