The following program demonstrates an Example of Using Base Keyword in C#.
Basically, the base keyword in C# refers to the method or constructor of the base class. When we need to explicitly call a particular constructor of the base class from a constructor in the subclass, we can use the base keyword. The following program shows how to call the base class constructor from the subclass constructor.
Another use of the base keyword is in calling the superclass method which has the same name as a method in the subclass. The following program shows how the show() method of the base class Person is called within the show() method of the subclass Customer.
using System;
namespace BaseKeywordDemo
{
class Program
{
static void Main(string[] args)
{
Customer ob1, ob2, ob3;
ob1 = new Customer();
ob1.show();
ob2 = new Customer("NoteBook", 50);
ob2.show();
ob3 = new Customer(ob2);
ob3.show();
}
}
class Person
{
protected int id_no;
protected String name;
int age;
public Person()
{
id_no = 1;
name = "";
age = 1;
Console.WriteLine("Inside Default Constructor of Person class!");
}
public Person(int id_no, String name, int age)
{
this.id_no = id_no;
this.name = name;
this.age = age;
Console.WriteLine("Inside Parameterized Constructor of Person class!");
}
public Person(Person ob)
{
id_no = ob.id_no;
name = ob.name;
age = ob.age;
Console.WriteLine("Inside Copy Constructor of Person class!");
}
public void show()
{
String str = "\nPerson ID: " + id_no + " Person Name: " + name + " Age: " + age;
Console.WriteLine(str);
}
}
class Customer: Person
{
String item_name;
int item_price;
public Customer():base(11, "ABC", 20)
{
item_name = "";
item_price = 0;
Console.WriteLine("Inside Default Constructor of Customer class!");
}
public Customer(String item_name, int price):base()
{
this.item_name = item_name;
this.item_price = price;
Console.WriteLine(item_name + " " + item_price);
Console.WriteLine("Inside Parameterized Constructor of Customer class!");
}
public Customer(Customer ob) : base(12, "XYZ", 25)
{
item_name = ob.item_name;
item_price = ob.item_price;
Console.WriteLine("Inside Copy Constructor of Customer class!");
}
public void show()
{
base.show();
String str = "Item Name: " + item_name + " Item Price: " + item_price;
Console.WriteLine("\n" + str);
}
}
}
Output
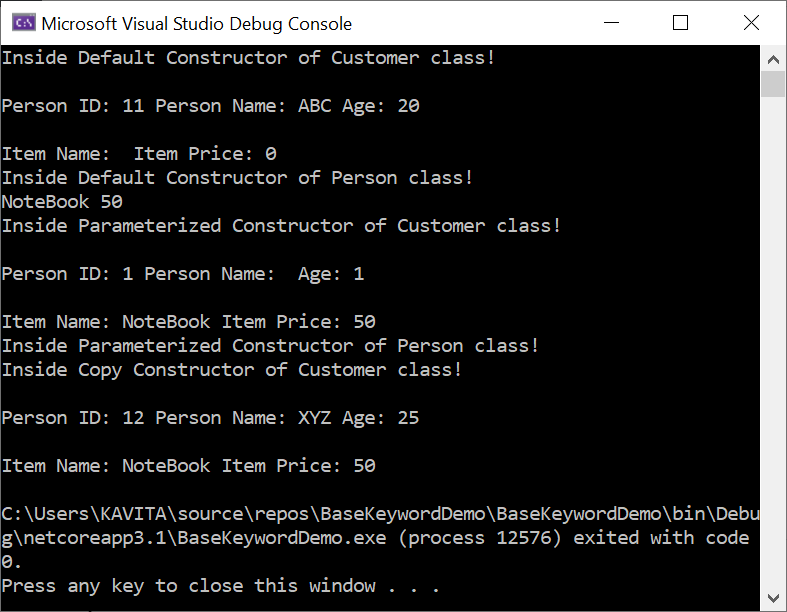
Further Reading
How to Create Instance Variables and Class Variables in Python
Comparing Rows of Two Tables with ADO.NET
Example of Label and Textbox Control in ASP.NET
One Dimensional and Two Dimensuonal Indexers in C#
Private and Static Constructors in C#
- Angular
- ASP.NET
- Bootstrap
- C
- C#
- C++
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
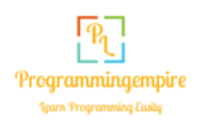