The following program demonstrates an Example of Computed Property in C#.
Basically, the value of a computed property is computed each time its getter is called. Moreover, a computed property doesn’t map to a field in the class. The following program demonstrates a computed property called Area.
using System;
namespace ComputedPropertyExample
{
class Circle
{
const double pi = 3.14;
//Read-Write Property
public double Radius { get; set; } = 5.778;
public double Area
{
get
{
return pi * Radius * Radius;
}
}
}
class Program
{
static void Main(string[] args)
{
Circle c = new Circle();
Circle c1 = new Circle { Radius = 2.11 };
//Display Computed Properties
Console.WriteLine($"Area = {c.Area}");
Console.WriteLine($"Area = {c1.Area}");
}
}
}
Output
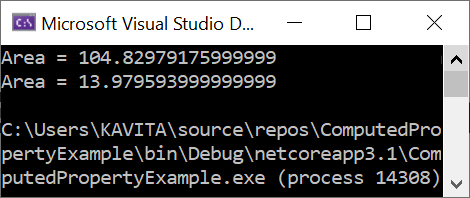
Further Reading
How to Create Instance Variables and Class Variables in Python
Comparing Rows of Two Tables with ADO.NET
Example of Label and Textbox Control in ASP.NET
One Dimensional and Two Dimensuonal Indexers in C#
Private and Static Constructors in C#
- Angular
- ASP.NET
- Bootstrap
- C
- C#
- C++
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
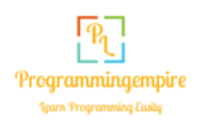