The following code shows an Example of Abstract Method in Java.
Problem Statement
Further, Implement the above program using abstract classes by making the Figure class abstract.
Solution
// Abstract Figure class
abstract class Figure {
// Abstract method for calculating area (to be implemented by subclasses)
abstract double calculateArea();
}
// Rectangle subclass
class Rectangle extends Figure {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
// Implement the abstract method to calculate the area of a rectangle
@Override
double calculateArea() {
return length * width;
}
}
// Triangle subclass
class Triangle extends Figure {
private double base;
private double height;
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
// Implement the abstract method to calculate the area of a triangle
@Override
double calculateArea() {
return 0.5 * base * height;
}
}
public class AreaCalculator {
public static void main(String[] args) {
// Create objects of Rectangle and Triangle
Figure rectangle = new Rectangle(5.0, 3.0);
Figure triangle = new Triangle(4.0, 6.0);
// Calculate and display the area using dynamic method dispatch
System.out.println("Area of the Rectangle: " + rectangle.calculateArea());
System.out.println("Area of the Triangle: " + triangle.calculateArea());
}
}
In this program:
- We have an abstract class
Figure
with an abstract methodcalculateArea()
. This method will be implemented by the subclasses. - The
Rectangle
andTriangle
classes are subclasses ofFigure
. They implement thecalculateArea()
method to calculate the area specific to each shape. - In the
main
method, we create objects of theRectangle
andTriangle
classes and store them in references of theFigure
type. This allows us to use dynamic method dispatch to call the appropriatecalculateArea()
method based on the actual object type. - We calculate and display the areas of the rectangle and triangle using dynamic method dispatch.
Using abstract classes and method overriding, this program demonstrates the concept of polymorphism and dynamic method dispatch to calculate the areas of different shapes.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
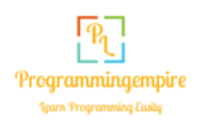