This article demonstrates a Dynamic Method Dispatch Example in Java.
Problem Statement
Write a program in java to Create a class Figure and its 2 subclasses Rectangle and Triangle and calculate its area using dynamic method dispatch.
Solution
class Figure {
double calculateArea() {
return 0.0;
}
}
class Rectangle extends Figure {
double length;
double width;
Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
@Override
double calculateArea() {
return length * width;
}
}
class Triangle extends Figure {
double base;
double height;
Triangle(double base, double height) {
this.base = base;
this.height = height;
}
@Override
double calculateArea() {
return 0.5 * base * height;
}
}
public class Main {
public static void main(String[] args) {
Figure[] figures = new Figure[3];
figures[0] = new Rectangle(5.0, 4.0);
figures[1] = new Triangle(6.0, 8.0);
figures[2] = new Rectangle(3.0, 7.0);
for (Figure figure : figures) {
System.out.println("Area: " + figure.calculateArea());
}
}
}
In this program:
- We have a base class
Figure
with a methodcalculateArea()
that returns 0.0 by default. - Two subclasses,
Rectangle
andTriangle
, inherit fromFigure
and override thecalculateArea()
method to calculate the area specific to each figure. - In the
main
method, we create an array ofFigure
objects, which can hold objects of both the base class and its subclasses. - We create instances of
Rectangle
andTriangle
and store them in thefigures
array. - Finally, we use a for loop to iterate through the
figures
array and call thecalculateArea()
method for each figure. Dynamic method dispatch ensures that the correctcalculateArea()
method (either fromRectangle
orTriangle
) is called based on the actual object type.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
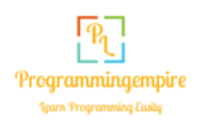