The following program demonstrates an Example of a Custom Exception in C#.
In this program, a custom exception class TempIsZeroException is created. In order to create a custom exception class, we must inherit it from the Exception class. Also, note the use of the throw keyword, which manually throws an exception. When the variable temperature becomes zero, the custom exception is thrown.
using System;
namespace CustomExceptionDemo
{
class Program
{
static void Main(string[] args)
{
Temperature temp = new Temperature();
try
{
temp.showTemp();
}
catch (TempIsZeroException e)
{
Console.WriteLine("TempIsZeroException: {0}", e.Message);
}
}
}
public class TempIsZeroException : Exception
{
public TempIsZeroException(string message) : base(message)
{
}
}
public class Temperature
{
int temperature = 0;
public void showTemp()
{
if (temperature == 0)
{
throw (new TempIsZeroException("Zero Temperature found"));
}
else
{
Console.WriteLine("Temperature: {0}", temperature);
}
}
}
}
Output
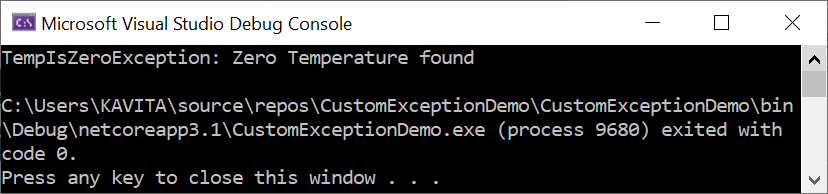
Further Reading
How to Create Instance Variables and Class Variables in Python
Comparing Rows of Two Tables with ADO.NET
Example of Label and Textbox Control in ASP.NET
One Dimensional and Two Dimensuonal Indexers in C#
Private and Static Constructors in C#
- Angular
- ASP.NET
- Bootstrap
- C
- C#
- C++
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
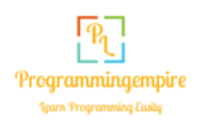