The following code shows how to Create an Array and Display it in JavaScript. As can be seen in the following code, we create an array by taking initial values. In order to initialize an array, a comma-separated list of values enclosed in square brackets is used. Further, the index starts with 0. So, the last index is one less than the total number of elements. Also, we use here the length property of an array that represents the total number of elements in the array.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
var arr1=[1,2,3,4,5];
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
</script>
</head>
<body>
</body>
</html>
Output
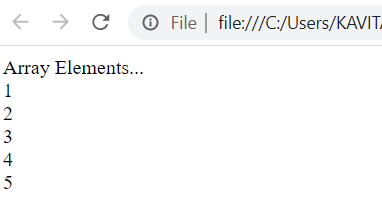
The following code shows how to create an array using the Array constructor. When we use this method, we can assign initial values to the array elements. Furthermore, a single value within the parenthesis represents the size of the array. For instance, Array(5) represents an array of five undefined elements.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
var arr1=Array(11,12,13,14);
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
</script>
</head>
<body>
</body>
</html>
Output
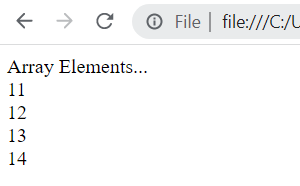
The following code shows creating an array by taking user input. Evidently, here we use the Array() constructor to create an array of a specific size. So, the variable arr1 represents an array of three undefined elements. Further, we use a loop to accept the input from the user. Basically, the prompt() function takes an input from the user and returns it as a string. Therefore, we need to convert it into a numeric type to use the value in an arithmetic expression. Hence, we use the Number() method to convert the input values into numeric type values. Moreover, JavaScript array can take elements of different types.
<html>
<head>
<title>JavaScript Arrays</title>
<script>
//An array of length 3
var arr1=Array(3);
for(var i=0;i<arr1.length;i++)
{
var x=prompt("Enter array element: ");
arr1[i]=Number(x);
}
document.write("Array Elements...<br/>");
for(var i=0;i<arr1.length;i++)
document.write(arr1[i]+"<br/>");
</script>
</head>
<body>
</body>
</html>
Output
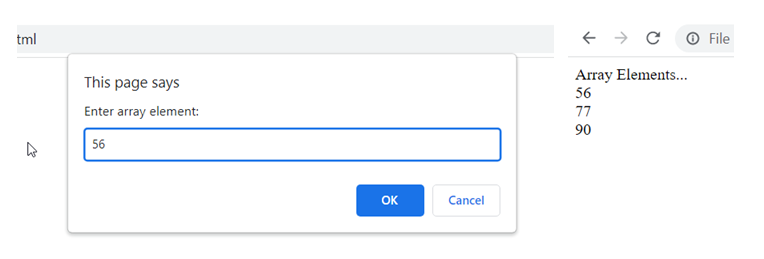
Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
Creating Classes in JavaScript
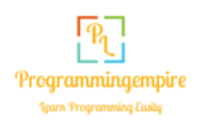