The following program demonstrates how to Find Distinct Elements in a Set in C#.
In order to find the distinct elements in an array, we use the Distinct() method of LINQ (Language Integrated Query). Basically, the Distinct() method works on a data source. So, we take the array containing duplicate elements as the data source. Further, the method returns the distinct elements from the data source.
using System;
using System.Linq;
namespace DistinctMethodDemo
{
class Program
{
static void Main(string[] args)
{
int[] arr = {1,2,2,1,1,1,1,3,4,1,2,3,3,4,7,5,6,6,5,8,9,12,14,15,1,2,8,5,70,5,5,6,16 };
Console.WriteLine("All Elements in the Set...");
foreach (int i in arr)
Console.Write(i + " ");
Console.WriteLine();
Console.WriteLine("Distinct Elements in the Set...");
var q = arr.Distinct();
foreach (int i in q)
Console.Write(i + " ");
Console.WriteLine();
}
}
}
Output
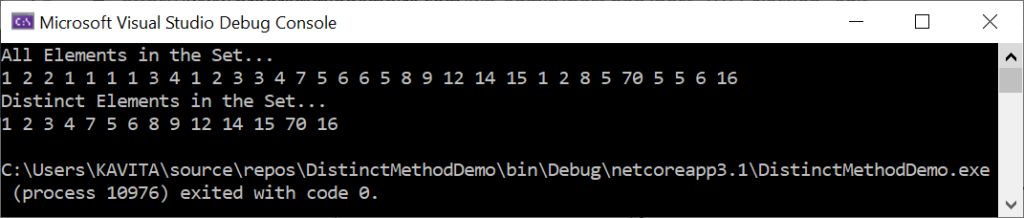
Further Reading
How to Create Instance Variables and Class Variables in Python
Comparing Rows of Two Tables with ADO.NET
Example of Label and Textbox Control in ASP.NET
One Dimensional and Two Dimensuonal Indexers in C#
Private and Static Constructors in C#
- Angular
- ASP.NET
- Bootstrap
- C
- C#
- C++
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
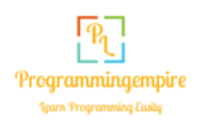