The following article describes When and how to use HashMap in Java.
Basically, HashMap class implements the Map interface. Furthermore, the Map interface is available in the java.util package. We use the HashMap class to store the key-value pairs. In a key-value pair each unique key maps to a corresponding value. In fact, HashMap provides constant-time performance for the basic operations (get and put). Therefore, it makes it an efficient data structure for many use cases.
The following list specifies some common scenarios where you might use a HashMap
.
- When you need to associate values with keys, such as in a dictionary.
- In case you need to keep track of counts, such as counting the frequency of words in a document.
- Moreover, you can use hashMap when you need to implement a cache where you store frequently accessed data, mapping keys to values.
The following example demonstrates the use of a HashMap
in Java.
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
// add key-value pairs to the map
map.put("Luke", 65);
map.put("Tane", 80);
map.put("Reem", 45);
// retrieve a value based on its key
int age = map.get("Luke");
System.out.println("Luke's age: " + age);
// check if a key is present in the map
if (map.containsKey("Reem")) {
System.out.println("Reem found on the map.");
}
}
}
Note that the HashMap class is not synchronized, so if you need to use it in a multi-threaded environment, you may want to use the synchronized java.util.Collections.synchronizedMap
method to create a synchronized HashMap
.
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
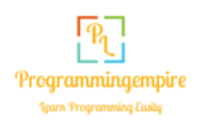