The following code demonstrates ten String Class Methods in Java.
Problem Statement
Create a Java application to demonstrate the working of any Ten String class functions. Also, Write code to extract the surname from the complete name
public class StringFunctionsDemo {
public static void main(String[] args) {
// Sample input string
String fullName = "John Doe";
// 1. Length of the string
int length = fullName.length();
System.out.println("1. Length of the full name: " + length);
// 2. Convert to uppercase
String upperCaseFullName = fullName.toUpperCase();
System.out.println("2. Uppercase: " + upperCaseFullName);
// 3. Convert to lowercase
String lowerCaseFullName = fullName.toLowerCase();
System.out.println("3. Lowercase: " + lowerCaseFullName);
// 4. Check if it starts with a specific prefix
boolean startsWithJohn = fullName.startsWith("John");
System.out.println("4. Starts with 'John': " + startsWithJohn);
// 5. Check if it ends with a specific suffix
boolean endsWithDoe = fullName.endsWith("Doe");
System.out.println("5. Ends with 'Doe': " + endsWithDoe);
// 6. Replace a substring
String replacedFullName = fullName.replace("John", "Jane");
System.out.println("6. Replaced 'John' with 'Jane': " + replacedFullName);
// 7. Get the character at a specific index
char firstChar = fullName.charAt(0);
System.out.println("7. First character: " + firstChar);
// 8. Split the name into first and last name
String[] nameParts = fullName.split(" ");
String firstName = nameParts[0];
String lastName = nameParts[1];
System.out.println("8. First Name: " + firstName);
System.out.println(" Last Name: " + lastName);
// 9. Check if it contains a specific substring
boolean containsDoe = fullName.contains("Doe");
System.out.println("9. Contains 'Doe': " + containsDoe);
// 10. Extract surname from the full name
String[] fullNameParts = fullName.split(" ");
if (fullNameParts.length >= 2) {
String surname = fullNameParts[1];
System.out.println("10. Surname: " + surname);
} else {
System.out.println("10. Invalid full name format.");
}
}
}
In this Java program, we demonstrate the following string functions.
length()
: Gets the length of the string.toUpperCase()
: Converts the string to uppercase.toLowerCase()
: Converts the string to lowercase.startsWith()
: Checks if the string starts with a specific prefix.endsWith()
: Checks if the string ends with a specific suffix.replace()
: Replaces a substring with another substring.charAt()
: Gets the character at a specific index.split()
: Splits the string into substrings using a delimiter.contains()
: Checks if the string contains a specific substring.- Custom code to extract the surname from a full name.
Compile and run this Java program to see the output demonstrating these string functions, including the extraction of the surname from the full name.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
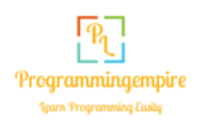