The following example code demonstrates how to Create a JSP Filter that Prints the IP Address.
Here is an example code for a JSP filter that prints the IP address of the client.
- IpFilter.java
import java.io.IOException;
import javax.servlet.*;
import javax.servlet.annotation.WebFilter;
@WebFilter("/*")
public class IpFilter implements Filter {
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
String ipAddress = request.getRemoteAddr();
System.out.println("IP address: " + ipAddress);
chain.doFilter(request, response);
}
public void init(FilterConfig fConfig) throws ServletException {}
public void destroy() {}
}
- web.xml
<web-app>
<filter>
<filter-name>IpFilter</filter-name>
<filter-class>IpFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>IpFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
This code creates a JSP filter named IpFilter
that implements the Filter
interface. The doFilter
method is called every time a request is made to the web application. In this method, the client’s IP address is retrieved using request.getRemoteAddr()
and printed to the console. The filter is applied to all URLs in the web application by specifying /*
as the url-pattern
in the web.xml
configuration file.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
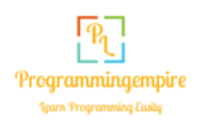