The following example demonstrates How to Use Two-Dimensional Array in C#.
Here we use a 2D array as a matrix. The following program shows how to input elements in a 2D array as well as display it as a matrix. Further, the program finds the sum of two matrices.
using System;
namespace TwoDArrayExample
{
class Program
{
static void Main(string[] args)
{
//program to find sum of two matrix;
//Declation of 2D array
int[,] m1 = new int[2, 2];
int[,] m2 = new int[2, 2];
int[,] m3 = new int[2, 2];
Console.WriteLine("Enter elements of first matrix: ");
for(int i=0;i<2;i++)
{
for(int j=0;j<2;j++)
{
m1[i, j] = Int32.Parse(Console.ReadLine());
}
}
Console.WriteLine("Enter elements of second matrix: ");
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 2; j++)
{
m2[i, j] = Int32.Parse(Console.ReadLine());
}
}
//Find Sum
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 2; j++)
{
m3[i, j] = m1[i, j] + m2[i, j];
}
}
Console.WriteLine("M1");
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 2; j++)
{
Console.Write(m1[i, j] + " ");
}
Console.WriteLine();
}
Console.WriteLine("M2");
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 2; j++)
{
Console.Write(m2[i, j] + " ");
}
Console.WriteLine();
}
Console.WriteLine("Sum matrix M3");
for (int i = 0; i < 2; i++)
{
for (int j = 0; j < 2; j++)
{
Console.Write(m3[i, j] + " ");
}
Console.WriteLine();
}
}
}
}
Output
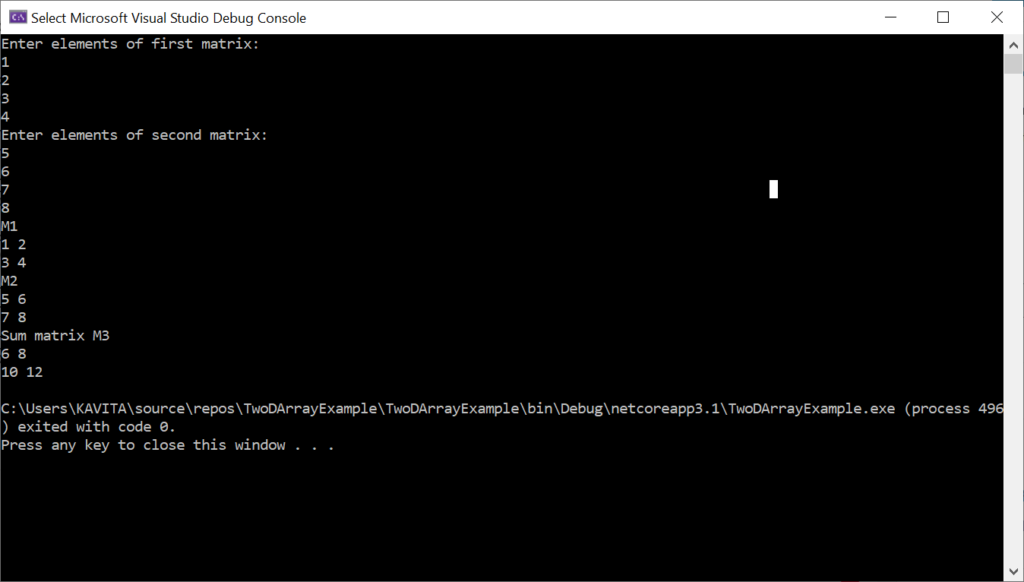
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
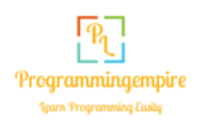