The following article explains How to Use Servlet Filters.
Basically, servlet filters are Java classes that can intercept incoming requests and outgoing responses to the server, allowing developers to perform pre- and post-processing on the request/response data.
The following code example demonstrates how servlet filters work.
- At first, create a new Java class that implements the javax.servlet.Filter interface.
import java.io.IOException;
import javax.servlet.*;
public class ExampleFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
// Perform some pre-processing on the request data here, if necessary
chain.doFilter(request, response);
// Perform some post-processing on the response data here, if necessary
}
@Override
public void init(FilterConfig filterConfig) throws ServletException {
// Initialization code goes here (optional)
}
@Override
public void destroy() {
// Cleanup code goes here (optional)
}
}
- Then, map the filter to a servlet or URL pattern in the web.xml deployment descriptor file.
<web-app>
...
<filter>
<filter-name>ExampleFilter</filter-name>
<filter-class>com.example.ExampleFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>ExampleFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
...
</web-app>
- Now, every time a client sends a request to the server, the ExampleFilter’s doFilter() method will be called before the request is processed by any servlets that match the URL pattern specified in the filter-mapping element.
In fact, the chain.doFilter(request, response) line tells the server to continue processing the request and pass it along to the next filter or servlet in the chain, or to send the response back to the client if there are no more filters or servlets to process.
- If the servlet that the filter is mapped to generates a response, the ExampleFilter’s doFilter() method will also be called again as part of the response processing phase.
Hence, it allows you to perform any necessary post-processing on the response data before it is sent back to the client.
That’s it! With this simple example, you can see how servlet filters can be used to intercept requests and responses and perform pre- and post-processing on the data.
The following example shows another dynamic web application using servlet filters.
Create a Dynamic Web Application that makes use of Servlet Filters
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
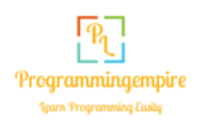