The following article demonstrates an Example of Using Servlet Filters in a Dynamic Web Application.
In order to create a Dynamic Web Application that makes use of Servlet Filters, follow these steps.
- Firstly, open your IDE (e.g. Eclipse, IntelliJ, etc.) and create a new Dynamic Web Application project. Give it a name and select the appropriate web server runtime.
- Then, create a new Servlet Filter by right-clicking on the project name in Package Explorer and selecting New > Servlet Filter. Give it a name (e.g. LoggingFilter) and click Finish.
- After that, edit the LoggingFilter class to perform some pre-processing on the request data and post-processing on the response data. The following code shows an example.
import java.io.IOException;
import java.util.logging.Logger;
import javax.servlet.*;
public class LoggingFilter implements Filter {
private static final Logger logger = Logger.getLogger(LoggingFilter.class.getName());
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
// Log the incoming request
logger.info("Received request: " + request.toString());
chain.doFilter(request, response);
// Log the outgoing response
logger.info("Sent response: " + response.toString());
}
@Override
public void init(FilterConfig filterConfig) throws ServletException {
// Initialization code goes here (optional)
}
@Override
public void destroy() {
// Cleanup code goes here (optional)
}
}
- Map the filter to a servlet or URL pattern in the web.xml deployment descriptor file. The following code shows it.
<web-app>
...
<filter>
<filter-name>LoggingFilter</filter-name>
<filter-class>com.example.LoggingFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>LoggingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
...
</web-app>
This maps the LoggingFilter to all requests that come into the server.
- Create a servlet that will respond to a request. The following code demonstrates it.
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class MyServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.getWriter().write("Hello, world!");
}
}
- After that, map the servlet to a URL pattern in the web.xml deployment descriptor file. The following example shows it.
<web-app>
...
<servlet>
<servlet-name>MyServlet</servlet-name>
<servlet-class>com.example.MyServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>MyServlet</servlet-name>
<url-pattern>/hello</url-pattern>
</servlet-mapping>
...
</web-app>
This maps the MyServlet to the “/hello” URL.
- Finally, deploy the application to your web server and access the URL in a web browser. You should see the “Hello, world!” message displayed in the browser.
Then, check the logs (e.g. in the console or a log file) and you should see log messages generated by the LoggingFilter for the incoming request and outgoing responses.
That’s it! You have created a Dynamic Web Application that makes use of a Servlet Filter.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
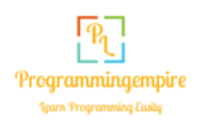