The following example code demonstrates How to Use sendRedirect() Method in a Servlet.
Here is an example of a dynamic web application using servlets to demonstrate the sendRedirect()
method.
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class RedirectServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String name = request.getParameter("name");
if (name == null || name.isEmpty()) {
response.sendRedirect("/RedirectedServlet?message=Please enter a name");
} else {
response.sendRedirect("/RedirectedServlet?message=Hello " + name);
}
}
}
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class RedirectedServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html>");
out.println("<head>");
out.println("<title>Redirected Servlet</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>Redirected Servlet</h1>");
String message = request.getParameter("message");
out.println("<p>" + message + "</p>");
out.println("</body>");
out.println("</html>");
}
}
In this example, the RedirectServlet
class handles GET requests and generates a redirect response. The redirect response causes the browser to make a new request for the specified URL. The sendRedirect()
method is used to generate the redirect response. If the name
parameter is not provided or is empty, the sendRedirect()
method is called with a URL that includes a message
parameter with the value “Please enter a name”. If the name
parameter is provided, the sendRedirect()
method is called with a URL that includes a message
parameter with the value “Hello ” followed by the name
parameter.
The RedirectedServlet
class handles GET requests for the redirected URL and generates an HTML response that includes the message
parameter in the body of the response. The sendRedirect()
method is useful for cases where a servlet needs to redirect the client to a different URL, such as in cases where authentication is required or in cases where the desired content is located at a different URL. When the sendRedirect()
method is called, the specified URL is loaded in the browser, effectively replacing the current URL.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
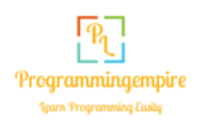