The following program demonstrates How to Use Layout Manager in Java.
Problem Statement
Develop an application in Java that creates 3 control boxes for font name, size, and style, change the font of the text according to user selection.
Solution
In Java, you can use layout managers to add different controls (components) to a frame in a specific arrangement. The following code shows an example of creating a Java program with a FlowLayout
layout manager to add different controls (buttons and labels) to a frame.
import java.awt.*;
import javax.swing.*;
public class LayoutManagerDemo {
public static void main(String[] args) {
// Create a JFrame
JFrame frame = new JFrame("Layout Manager Demo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 150);
// Create a JPanel with FlowLayout
JPanel panel = new JPanel(new FlowLayout());
// Add controls to the panel
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
JLabel label = new JLabel("Label");
panel.add(button1);
panel.add(button2);
panel.add(label);
// Add the panel to the frame
frame.add(panel);
// Make the frame visible
frame.setVisible(true);
}
}
In this program:
- We create a
JFrame
namedframe
and set its title, close operation, and size. - Then, we create a
JPanel
namedpanel
and set its layout manager toFlowLayout
. This means that any components added to this panel will be arranged in a flow from left to right, wrapping to the next line when the horizontal space is exhausted. - After that, we create and add three components to the
panel
: two buttons (button1
andbutton2
) and a label (label
). - Finally, we add the
panel
to theframe
and make theframe
visible.
When you run this program, you’ll see a JFrame
with a FlowLayout
layout manager that arranges the buttons and label horizontally. You can experiment with other layout managers, such as BorderLayout
, GridLayout
, or BoxLayout
, to achieve different component arrangements in your GUI.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
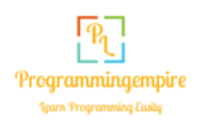