The following code demonstrates How to Use Graphics in AWT.
Problem Statement
Develop a Java application that shows working with Graphics, colors, and fonts in AWT.
Solution
Creating a Java application that demonstrates working with Graphics, colors, and fonts in AWT involves using the Graphics
class to draw shapes, apply colors, and set fonts. The following code shows an example of a Java application that showcases these concepts.
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class GraphicsDemo extends JFrame {
public GraphicsDemo() {
setTitle("Graphics, Colors, and Fonts Demo");
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
add(new DrawingPanel());
setVisible(true);
}
class DrawingPanel extends JPanel {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// Set background color
setBackground(Color.LIGHT_GRAY);
// Set font and draw text
Font font = new Font("Arial", Font.BOLD, 20);
g.setFont(font);
g.setColor(Color.BLUE);
g.drawString("Graphics Demo", 100, 30);
// Draw shapes
g.setColor(Color.RED);
g.drawRect(50, 50, 100, 50); // Rectangle
g.setColor(Color.GREEN);
g.fillOval(200, 50, 80, 80); // Filled oval
g.setColor(Color.ORANGE);
g.drawLine(50, 200, 250, 200); // Line
// Draw a custom shape
g.setColor(Color.MAGENTA);
int[] xPoints = {150, 200, 100};
int[] yPoints = {250, 300, 300};
int numPoints = 3;
g.fillPolygon(xPoints, yPoints, numPoints);
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new GraphicsDemo();
}
});
}
}
In this program:
- We create a
GraphicsDemo
class that extendsJFrame
to create the main window. - Inside the constructor:
- We set the title, size, close operation, and add a custom
DrawingPanel
to the frame.
- We set the title, size, close operation, and add a custom
- The
DrawingPanel
class is an inner class that extendsJPanel
and overrides itspaintComponent
method. Inside this method, we:- Set the background color of the panel to light gray.
- Also, set the font and draw text using
drawString
. - Draw various shapes such as rectangles, ovals, lines, and a custom polygon using different colors.
- Then, we set the main background color of the panel to light gray using
setBackground
. - In the
main
method, we useSwingUtilities.invokeLater
to create an instance of theGraphicsDemo
on the Swing Event Dispatch Thread.
When you run this program, you’ll see a GUI window displaying text and various shapes, demonstrating the use of graphics, colors, and fonts in AWT.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
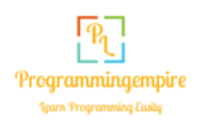