The following program demonstrates How to Use Event Handlers in Java.
In Java, you can use different event handlers to respond to various user interactions in a graphical user interface (GUI) application. The following code shows a Java program that demonstrates the use of different event handlers, including ActionListener, MouseListener, and KeyListener.
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class EventHandlersDemo {
private JFrame frame;
private JButton button;
private JTextField textField;
private JLabel label;
private JTextArea textArea;
public EventHandlersDemo() {
frame = new JFrame("Event Handlers Demo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 300);
button = new JButton("Click Me");
textField = new JTextField(20);
label = new JLabel("This is a label.");
textArea = new JTextArea(5, 30);
// ActionListener for the button
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String buttonText = button.getText();
label.setText("Button Clicked: " + buttonText);
}
});
// MouseListener for the label
label.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
label.setText("Label Clicked");
}
});
// KeyListener for the text field
textField.addKeyListener(new KeyAdapter() {
public void keyTyped(KeyEvent e) {
char keyChar = e.getKeyChar();
textArea.append("Key Typed: " + keyChar + "\n");
}
});
// Add components to the frame
frame.setLayout(new FlowLayout());
frame.add(button);
frame.add(textField);
frame.add(label);
frame.add(textArea);
frame.setVisible(true);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new EventHandlersDemo();
}
});
}
}
In this program:
- We create a
JFrame
and set its properties, including title, close operation, and size. - Then, we create various Swing components, such as
JButton
,JTextField
,JLabel
, andJTextArea
. - Next, we add event handlers to these components as follows:
- For the
button
, we add anActionListener
that responds when the button is clicked. - Similarly, for the
label
, we add aMouseListener
to detect mouse clicks. - Likewise, for the
textField
, we add aKeyListener
to capture key events when typing.
- For the
- Inside each event handler, we perform actions such as updating labels, displaying messages in a text area, or responding to user input.
- After that, we add all the components to the frame and set the frame’s layout to
FlowLayout
. - Finally, we make the frame visible.
When you run this program, you can interact with the GUI components and observe how different event handlers respond to button clicks, label clicks, and key typing events.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
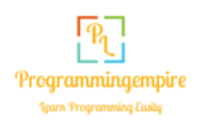