The following code example demonstrates How to Implement Stack in Java Using Interfaces.
In Java, you can implement a stack using interfaces by defining a custom interface for the stack and then creating a class that implements that interface. Here’s a step-by-step guide to implementing a stack using interfaces in Java.
Define a Stack interface
To begin with, create an interface that defines the basic operations of a stack, such as push, pop, peek, isEmpty, and size. For example.
public interface Stack<T> {
void push(T item);
T pop();
T peek();
boolean isEmpty();
int size();
}
In this example, we use a generic type T
to make the stack flexible and capable of storing different types of elements.
Implement the Stack interface
Once you define the Stack interface, we can create a class that implements the Stack
interface. Also, you will need to provide the concrete implementation for each of the methods defined in the interface. For instance, given below is an example implementation using an array-based approach.
public class ArrayStack<T> implements Stack<T> {
private static final int DEFAULT_CAPACITY = 10;
private Object[] elements;
private int size;
public ArrayStack() {
elements = new Object[DEFAULT_CAPACITY];
size = 0;
}
@Override
public void push(T item) {
if (size == elements.length) {
resizeArray();
}
elements[size++] = item;
}
@Override
public T pop() {
if (isEmpty()) {
throw new IllegalStateException("Stack is empty");
}
T item = peek();
elements[--size] = null; // Clear the reference to the popped item
return item;
}
@Override
public T peek() {
if (isEmpty()) {
throw new IllegalStateException("Stack is empty");
}
return (T) elements[size - 1];
}
@Override
public boolean isEmpty() {
return size == 0;
}
@Override
public int size() {
return size;
}
private void resizeArray() {
int newCapacity = elements.length * 2;
elements = Arrays.copyOf(elements, newCapacity);
}
}
In this implementation, we use an array to store stack elements and the resizeArray
method is called when the array is full to double its capacity.
Using the Stack implementation
You can now use the ArrayStack
class or any other class that implements the Stack
interface to work with stacks in your Java program. The following code shows an example of how to use it.
public class Main {
public static void main(String[] args) {
Stack<Integer> stack = new ArrayStack<>();
stack.push(1);
stack.push(2);
stack.push(3);
System.out.println("Stack size: " + stack.size()); // Output: Stack size: 3
System.out.println("Top element: " + stack.peek()); // Output: Top element: 3
while (!stack.isEmpty()) {
System.out.println("Popped: " + stack.pop());
}
}
}
This code demonstrates how to create a stack, push elements onto it, check its size, peek at the top element, and pop elements from it.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
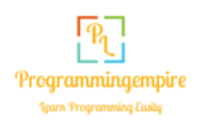