The following program demonstrates How to Display the Hostname for an IP Address in Java.
You can create a Java application that accepts a hostname and displays its IP address using the InetAddress
class from the java.net
package. The following code shows a simple example.
import java.net.InetAddress;
import java.net.UnknownHostException;
import java.util.Scanner;
public class HostnameToIPAddress {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Prompt the user to enter a hostname
System.out.print("Enter a hostname: ");
String hostname = scanner.nextLine();
try {
// Get the IP address for the given hostname
InetAddress ipAddress = InetAddress.getByName(hostname);
// Display the IP address
System.out.println("IP Address for " + hostname + ": " + ipAddress.getHostAddress());
} catch (UnknownHostException e) {
System.err.println("Unable to resolve the hostname: " + e.getMessage());
}
scanner.close();
}
}
In this program:
- We import the necessary classes from the
java.net
package. - Then, we create a
Scanner
to accept user input. - After that, we prompt the user to enter a hostname.
- Inside a
try-catch
block, we useInetAddress.getByName(hostname)
to resolve the hostname to an IP address. This method may throw aUnknownHostException
if the hostname cannot be resolved. - If the hostname is successfully resolved, we use
ipAddress.getHostAddress()
to get the IP address and display it to the user. - Otherwise, if the hostname cannot be resolved, we catch the
UnknownHostException
and display an error message.
Now, you can compile and run this Java program. It will prompt you to enter a hostname, and then it will display the corresponding IP address.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
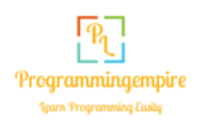