The following program demonstrates How to Create a Table in a Database in Java.
You can create a table in a database via Java using JDBC (Java Database Connectivity). Here’s an example of how to create a table in a MySQL database.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
public class CreateTableDemo {
public static void main(String[] args) {
// Database connection parameters
String url = "jdbc:mysql://localhost:3306/mydatabase";
String username = "your_username";
String password = "your_password";
// SQL statement to create a table
String createTableSql = "CREATE TABLE IF NOT EXISTS mytable ("
+ "id INT AUTO_INCREMENT PRIMARY KEY,"
+ "name VARCHAR(255),"
+ "age INT)";
try {
// Establish a database connection
Connection connection = DriverManager.getConnection(url, username, password);
// Create a statement
Statement statement = connection.createStatement();
// Execute the CREATE TABLE statement
statement.executeUpdate(createTableSql);
System.out.println("Table created successfully.");
// Close resources
statement.close();
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
In this example:
- Import the necessary JDBC classes.
- Specify the database connection parameters (URL, username, and password) to connect to your MySQL database.
- Define an SQL statement to create the table. The SQL statement includes the table name (
mytable
) and its columns (id
,name
, andage
). In this example, we create a simple table with an auto-increment primary key, a name column (VARCHAR), and an age column (INT). - Establish a database connection using
DriverManager.getConnection
. - Create a
Statement
to execute SQL statements. - Execute the
CREATE TABLE
statement usingexecuteUpdate
. TheIF NOT EXISTS
clause ensures that the table is created only if it doesn’t already exist. - Display a message indicating that the table has been created successfully.
- Close resources (statement and connection) in a
finally
block or using try-with-resources.
Make sure to replace the following placeholders with your own database information:
url
: The JDBC URL of your MySQL database.username
: Your database username.password
: Your database password.
Before running the program, ensure that your MySQL database server is running and that you have the appropriate permissions to create tables in the specified database.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
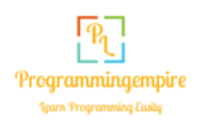