The following program demonstrates How to Create a Swing Login Window.
In order to create a Swing login window to check a username and password using text fields and command buttons, you can use the following Java program.
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class LoginWindow extends JFrame {
private JTextField usernameField;
private JPasswordField passwordField;
private JButton loginButton;
private JLabel resultLabel;
public LoginWindow() {
setTitle("Login Window");
setSize(300, 150);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(3, 2));
JLabel usernameLabel = new JLabel("Username:");
usernameField = new JTextField(20);
JLabel passwordLabel = new JLabel("Password:");
passwordField = new JPasswordField(20);
loginButton = new JButton("Login");
loginButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String username = usernameField.getText();
char[] passwordChars = passwordField.getPassword();
String password = new String(passwordChars);
if (isValidUser(username, password)) {
resultLabel.setText("Login Successful!");
} else {
resultLabel.setText("Invalid username or password. Please try again.");
}
}
});
resultLabel = new JLabel("");
panel.add(usernameLabel);
panel.add(usernameField);
panel.add(passwordLabel);
panel.add(passwordField);
panel.add(loginButton);
add(panel, BorderLayout.CENTER);
add(resultLabel, BorderLayout.SOUTH);
setVisible(true);
}
private boolean isValidUser(String username, String password) {
// Replace this with your actual authentication logic
// For simplicity, we're checking if the username is "user" and the password is "password"
return "user".equals(username) && "password".equals(password);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new LoginWindow();
}
});
}
}
In this program:
- We create a
LoginWindow
class that extendsJFrame
to create the main login window. - Inside the constructor:
- We set the title, size, and close operation for the frame.
- Then, we create Swing components such as
JLabel
,JTextField
,JPasswordField
,JButton
, andJLabel
for displaying the result. - Further, we add an
ActionListener
to the login button to validate the username and password when the button is clicked.
- The
isValidUser
method is used to simulate user authentication. You should replace it with your actual authentication logic. - Finally, we use
SwingUtilities.invokeLater
to create an instance of theLoginWindow
on the Swing Event Dispatch Thread.
When you run this program, you’ll see a Swing-based login window where users can enter a username and password. So, when the “Login” button is clicked, it checks the credentials and displays a message accordingly.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
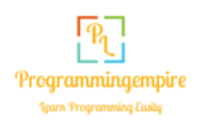