The following program demonstrates How to Change the Font of the Text According to User Selection in Java.
You can create a Java application that allows users to change the font name, size, and style of text using control boxes. The following code shows a simple example of such an application using Swing.
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class FontSelectorApp extends JFrame {
private JComboBox<String> fontComboBox;
private JComboBox<Integer> sizeComboBox;
private JCheckBox boldCheckBox;
private JCheckBox italicCheckBox;
private JTextArea textArea;
public FontSelectorApp() {
setTitle("Font Selector");
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
// Create control boxes
JPanel controlPanel = new JPanel();
fontComboBox = new JComboBox<>(GraphicsEnvironment.getLocalGraphicsEnvironment().getAvailableFontFamilyNames());
sizeComboBox = new JComboBox<>(new Integer[]{12, 14, 16, 18, 20, 24, 28, 32});
boldCheckBox = new JCheckBox("Bold");
italicCheckBox = new JCheckBox("Italic");
controlPanel.add(new JLabel("Font:"));
controlPanel.add(fontComboBox);
controlPanel.add(new JLabel("Size:"));
controlPanel.add(sizeComboBox);
controlPanel.add(boldCheckBox);
controlPanel.add(italicCheckBox);
// Create text area
textArea = new JTextArea("Sample Text", 5, 30);
textArea.setFont(new Font("Arial", Font.PLAIN, 16));
add(controlPanel, BorderLayout.NORTH);
add(new JScrollPane(textArea), BorderLayout.CENTER);
// Add action listeners to control boxes
fontComboBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
updateFont();
}
});
sizeComboBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
updateFont();
}
});
boldCheckBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
updateFont();
}
});
italicCheckBox.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
updateFont();
}
});
setVisible(true);
}
private void updateFont() {
String fontName = fontComboBox.getSelectedItem().toString();
int fontSize = (int) sizeComboBox.getSelectedItem();
int fontStyle = Font.PLAIN;
if (boldCheckBox.isSelected()) {
fontStyle += Font.BOLD;
}
if (italicCheckBox.isSelected()) {
fontStyle += Font.ITALIC;
}
Font selectedFont = new Font(fontName, fontStyle, fontSize);
textArea.setFont(selectedFont);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new FontSelectorApp();
}
});
}
}
In this Java Swing application:
- At first, we create a
FontSelectorApp
class that extendsJFrame
to create the main window. - Inside the constructor:
- Next, we create control boxes for font name, size, and style using
JComboBox
andJCheckBox
components. - We create a
JTextArea
where the user can see the text with the selected font.
- Next, we create control boxes for font name, size, and style using
- Then, we add action listeners to the control boxes (
fontComboBox
,sizeComboBox
,boldCheckBox
, anditalicCheckBox
) so that when the user changes their selections, theupdateFont
method is called to update the font of the text in thetextArea
. - The
updateFont
method retrieves the selected font name, size, and style from the control boxes and sets the font of the text area accordingly. - In the
main
method, we useSwingUtilities.invokeLater
to create an instance of theFontSelectorApp
on the Swing Event Dispatch Thread.
When you run this application, you can select different font names, sizes, and styles to change the font of the displayed text.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
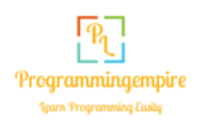