The following article demonstrates some Examples of Applets in Java.
In Java, applets are small applications that run within a web browser. They have a specific lifecycle defined by the Applet class. The lifecycle methods are called at various stages of an applet’s existence. The following code shows a simple applet program that demonstrates the applet lifecycle, followed by another program that shows the implementation of an applet.
Applet Lifecycle Program
import java.applet.Applet;
import java.awt.Graphics;
public class AppletLifecycleDemo extends Applet {
public void init() {
// Initialization code goes here
System.out.println("Applet initialized");
}
public void start() {
// Start code goes here
System.out.println("Applet started");
}
public void paint(Graphics g) {
// Drawing code goes here
g.drawString("Applet is running", 50, 50);
}
public void stop() {
// Stop code goes here
System.out.println("Applet stopped");
}
public void destroy() {
// Cleanup code goes here
System.out.println("Applet destroyed");
}
}
In this program:
init()
: This method is called when the applet is first initialized. It is used for one-time setup, such as initializing variables.start()
: This method is called when the applet is started. It is often used to start animations or other ongoing tasks.paint(Graphics g)
: This method is called whenever the applet needs to be redrawn. You can use it to draw graphics or display text.stop()
: This method is called when the applet is stopped, such as when the user navigates away from the applet’s web page.destroy()
: This method is called when the applet is about to be destroyed. It can be used for cleanup tasks.
Applet Implementation Example
Below is a basic HTML file that embeds the above applet.
<!DOCTYPE html>
<html>
<head>
<title>Applet Lifecycle Demo</title>
</head>
<body>
<applet code="AppletLifecycleDemo.class" width="300" height="200">
Your browser does not support Java applets.
</applet>
</body>
</html>
To run this applet:
- Save the Java program as
AppletLifecycleDemo.java
. - Compile it using
javac AppletLifecycleDemo.java
to generateAppletLifecycleDemo.class
. - Create an HTML file (e.g.,
applet.html
) with the above code and place it in the same directory as theAppletLifecycleDemo.class
file. - Open the HTML file in a web browser that supports Java applets.
When you run the HTML file, it will display the applet, and you’ll see messages in the Java console or terminal indicating the various stages of the applet lifecycle.
Please note that Java applets are no longer widely supported in modern web browsers due to security concerns. So, running this example may require you to use an older browser or a specialized environment.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
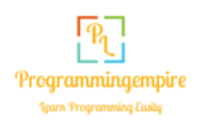