This article demonstrates an Example of Reference Variables in Java.
Problem Statement
Build a Java application to create a class called Test and pass its object to one of its methods to illustrate the usage of reference variables.
Solution
class Test {
int value;
// A method that takes an object of the Test class as a parameter
void modifyValue(Test obj) {
obj.value = 100;
}
}
public class ReferenceVariableDemo {
public static void main(String[] args) {
// Create an object of the Test class
Test testObject = new Test();
// Print the initial value
System.out.println("Initial value: " + testObject.value);
// Pass the object to the modifyValue method
testObject.modifyValue(testObject);
// Print the modified value
System.out.println("Modified value: " + testObject.value);
}
}
In this program:
- We define a class called
Test
with an instance variablevalue
and a methodmodifyValue
that takes an object of theTest
class as a parameter and modifies itsvalue
field. - In the
main
method, we create an objecttestObject
of theTest
class. - We print the initial value of the
value
field. - We pass the
testObject
to themodifyValue
method, which modifies thevalue
field of the object. - Finally, we print the modified value of the
value
field.
When you run this Java program, you’ll see that the object’s reference is passed to the modifyValue
method, and any changes made to the object within the method affect the original object. This demonstrates the usage of reference variables in Java.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
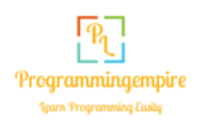