The following code shows an Example of Nested Interface in Java.
In Java, you can nest interfaces within other interfaces or classes. This allows you to create more structured and modular code. For instance, given below an example demonstrating how to nest interfaces in Java.
public class NestedInterfaceDemo {
// Outer interface
interface Shape {
void draw();
// Nested interface
interface Colorable {
void setColor(String color);
}
}
// Implementing the nested interface
static class Circle implements Shape, Shape.Colorable {
private String color;
@Override
public void draw() {
System.out.println("Drawing a circle");
}
@Override
public void setColor(String color) {
this.color = color;
}
public void printColor() {
System.out.println("Circle color: " + color);
}
}
public static void main(String[] args) {
Circle circle = new Circle();
circle.draw();
circle.setColor("Red");
circle.printColor();
}
}
In this example:
- We have an outer interface called
Shape
, which declares a methoddraw()
. Inside theShape
interface, there’s a nested interface calledColorable
, which declares a methodsetColor(String color)
. - Then, we have a class called
Circle
that implements both theShape
andShape.Colorable
interfaces. It provides concrete implementations for thedraw()
method from the outer interface and thesetColor(String color)
method from the nested interface. - In the
main
method, we create an instance of theCircle
class, call its methods to draw the circle, set its color, and print the color.
This demonstrates the nesting of interfaces in Java, where the nested interface Colorable
is accessible within the outer interface Shape
, and the class Circle
implements both the outer and nested interfaces.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
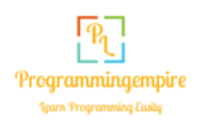