foExample of Multithreading in Java
Problem Statement
Write Java code to print multiples of 2,3,4 simultaneously.
Solution
In order to print multiples of 2, 3, and 4 simultaneously using threads in Java, you can create three separate threads, each responsible for printing multiples of one of these numbers. The following Java program demonstrates this.
class MultiplesPrinter extends Thread {
private int multiple;
public MultiplesPrinter(int multiple) {
this.multiple = multiple;
}
@Override
public void run() {
for (int i = 1; i <= 10; i++) {
System.out.println("Multiple of " + multiple + ": " + (i * multiple));
try {
Thread.sleep(200); // Sleep for 200 milliseconds
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class MultiplesPrintingApp {
public static void main(String[] args) {
// Create three threads to print multiples of 2, 3, and 4
MultiplesPrinter thread2 = new MultiplesPrinter(2);
MultiplesPrinter thread3 = new MultiplesPrinter(3);
MultiplesPrinter thread4 = new MultiplesPrinter(4);
// Start all three threads
thread2.start();
thread3.start();
thread4.start();
}
}
In this program:
- We create a
MultiplesPrinter
class that extends theThread
class. This class takes an integer parameter in its constructor, which represents the multiple to be printed (2, 3, or 4). - Inside the
run
method ofMultiplesPrinter
, we use a loop to print the multiples of the given number from 1 to 10. We also introduce a 200-millisecond delay between each print usingThread.sleep()
. - In the
main
method, we create three instances ofMultiplesPrinter
for multiples 2, 3, and 4. - We start all three threads using the
start()
method.
As a result, all three threads will run concurrently and print the multiples of 2, 3, and 4 simultaneously, creating an interleaved output.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
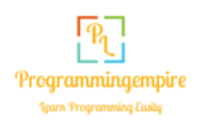