Programmingempire
The following code shows an Example of getElementsByTagName() Method in JavaScript.
Demonstrating an Example of getElementsByTagName() Method in JavaScript
As can be seen in the example, there are several kinds of tags on the HTML page. For instance, here we have <div>, <button>, and <br> tags. The following code shows that the getElementsByTagName() method retrieves all elements belonging to a particular tag. Further, we use the for loop to display all of these tags.
<html>
<head>
<title>getElementsByTagName Demo</title>
<script>
function f1()
{
var v1=document.getElementById("d2");
var x1=document.getElementsByTagName("DIV");
var x2=document.getElementsByTagName("BUTTON");
var x3=document.getElementsByTagName("BR");
str="Total Number of Div Tags: "+x1.length;
str+="<br/>Ids of all Div Elements: ";
for(i=0;i<x1.length;i=i+1)
str+=x1[i].id+" ";
str+="<br/>Total Number of Button Tags: "+x2.length;
str+="<br/>Ids of all Button Elements: : ";
for(i=0;i<x2.length;i=i+1)
str+=x2[i].id+" ";
str+="<br/>Total Number of BR Tags: "+x3.length;
str1=v1.innerHTML;
str1+="<br/>"+str;
v1.style.width='400px';
v1.style.display='block';
v1.innerHTML=str1;
}
</script>
<style>
.c1{
border: 4px solid #33ff99;
border-radius: 10px;
background-color: #45aa77;
color: #aaffff;
font-size: 20px;
text-align: center;
margin:5px;
padding: 30px;
}
.c2{
border: 6px inset #ff8844;
border-radius: 10px;
background-color: #ffdd44;
color: #ff5566;
font-size: 25px;
text-align: left;
margin:40px;
padding: 5px;
width: 600px;
}
</style>
</head>
<body>
<div id="d1" class="c2"><br/><br/>
Example of getElementsByTagName() Method
<br/><br/>
<button id="b1" class="c1">Button 1</button>
<button id="b2" class="c1">Button 2</button>
<button id="b3" class="c1">Button 3</button>
<br/><br/>
<button id="b4" class="c1">Button 4</button>
<button id="b5" class="c1">Button 5</button>
<button id="b6" class="c1">Button 6</button><br/><br/>
<button id="b7" class="c1" onclick="f1()">Get Tag Details in the Document</button>
<br/><br/>
<div class="c2" id="d2" style="display:none;"></div>
</div>
</body>
</html>
Output
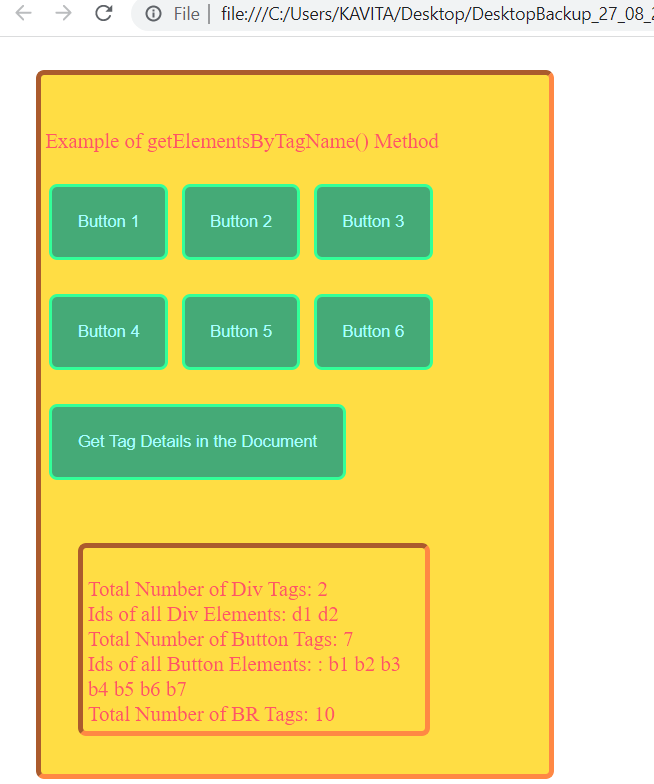
Further Reading
Evolution of JavaScript from ES1 to ES2020
Introduction to HTML DOM Methods in JavaScript
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
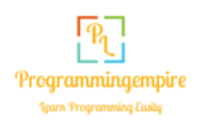