The following article demonstrates an Example of creating multiple contexts in Django.
In Django, you can create multiple contexts by passing multiple dictionaries to the render()
function when rendering a template. Here’s an example of how to create multiple contexts in Django.
- At first, create a new Django app inside your project.
python manage.py startapp myapp
- Then, create two new files called “base.html” and “home.html” inside your app’s “templates” directory, and add the following code.
base.html:
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}{% endblock %}</title>
</head>
<body>
<nav>
<a href="/">Home</a>
<a href="/about/">About</a>
</nav>
<main>
{% block content %}{% endblock %}
</main>
</body>
</html>
home.html:
{% extends "base.html" %}
{% block title %}{{ page_title }}{% endblock %}
{% block content %}
<h1>{{ page_heading }}</h1>
<p>{{ page_content }}</p>
{% endblock %}
This defines a base HTML template with a navigation menu and a content area, and a second template that extends the base template and provides content for the placeholders.
- Open the “views.py” file inside your app’s directory, and add the following code.
from django.shortcuts import render
def home_view(request):
context1 = {
'page_title': 'My Home Page',
'page_heading': 'Welcome to my home page',
'page_content': 'This is some example content',
}
context2 = {
'page_title': 'About Us',
'page_heading': 'About Our Company',
'page_content': 'Learn more about our company',
}
return render(request, 'home.html', {'context1': context1, 'context2': context2})
This defines a new view named “home_view” that creates two context dictionaries with values for the three placeholders in the template. It then calls the render()
function, passing in the request object, the name of the template to render, and a dictionary containing two keys, “context1” and “context2”, each with its own context dictionary as the value.
- Finally, add a URL pattern for your new view in your project’s “urls.py” file.
from django.urls import path
from myapp.views import home_view
urlpatterns = [
path('', home_view, name='home'),
]
This maps the URL “/” to your new view.
Now you can run your Django development server and visit the URL “/” to see your template in action! The two context dictionaries will be passed to the template, and the resulting HTML will contain content for both “context1” and “context2”.
Further Reading
Introduction to Django Framework and its Features
Examples of Array Functions in PHP
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
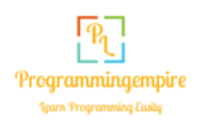