The following article demonstrates an Example of context variable lookup in Django.
In Django, context variable lookup is the process of accessing values from a context dictionary inside a template. Here’s an example of how to do a context variable lookup in Django.
- Create a new Django app inside your project.
python manage.py startapp myapp
- Create a new file called “home.html” inside your app’s “templates” directory, and add the following code:
<!DOCTYPE html>
<html>
<head>
<title>{{ page_title }}</title>
</head>
<body>
<h1>{{ page_heading }}</h1>
<p>{{ page_content }}</p>
{% if show_message %}
<p>{{ message }}</p>
{% endif %}
</body>
</html>
This is a simple HTML template with three placeholders for dynamic content: page_title
, page_heading
, and page_content
. It also includes a conditional block that only displays a message if a certain condition is met.
- Open the “views.py” file inside your app’s directory, and add the following code.
from django.shortcuts import render
def home_view(request):
context = {
'page_title': 'My Home Page',
'page_heading': 'Welcome to my home page',
'page_content': 'This is some example content',
'show_message': True,
'message': 'This is a special message!',
}
return render(request, 'home.html', context)
This defines a new view named “home_view” that creates a context dictionary with values for the three placeholders in the template, as well as two additional variables, “show_message” and “message”. The “show_message” variable is set to True
, so the message will be displayed in the template.
- Finally, add a URL pattern for your new view in your project’s “urls.py” file.
from django.urls import path
from myapp.views import home_view
urlpatterns = [
path('', home_view, name='home'),
]
This maps the URL “/” to your new view.
Now you can run your Django development server and visit the URL “/” to see your template in action! The placeholders in the template will be replaced with the values from the context dictionary, and the resulting HTML will contain the special message because the “show_message” variable is set to True
. If you set it to False
, the message will not be displayed.
Further Reading
Introduction to Django Framework and its Features
Examples of Array Functions in PHP
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
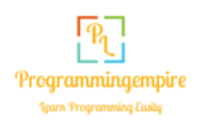