The following code demonstrates an Example of a Custom Exception in Java.
Problem Statement
Create an application that demonstrates how a program throws exceptions if the age of the voter is less than 18.
Solution
// Custom exception class for underage voters
class UnderageVoterException extends Exception {
public UnderageVoterException(String message) {
super(message);
}
}
// Voter class representing a voter's age
class Voter {
private int age;
public Voter(int age) {
this.age = age;
}
// Method to check if the voter is eligible
public void checkEligibility() throws UnderageVoterException {
if (age < 18) {
throw new UnderageVoterException("You must be 18 or older to vote.");
} else {
System.out.println("You are eligible to vote!");
}
}
}
// Main class to demonstrate the program
public class VoterAgeCheck {
public static void main(String[] args) {
try {
// Create a voter with age 16 (underage)
Voter underageVoter = new Voter(16);
// Check eligibility, may throw UnderageVoterException
underageVoter.checkEligibility();
} catch (UnderageVoterException e) {
System.out.println("Exception caught: " + e.getMessage());
}
}
}
In this program:
- We create a custom exception class
UnderageVoterException
that extendsException
to represent the scenario of an underage voter. - The
Voter
class contains anage
field and acheckEligibility
method. If the age of the voter is less than 18, it throws anUnderageVoterException
. Otherwise, it prints a message indicating eligibility. - In the
main
method, we create an instance of theVoter
class with an age of 16 (underage), and then we attempt to check their eligibility. Since the age is below 18, theUnderageVoterException
is thrown and caught in thecatch
block, where we print the exception message.
This program demonstrates how to throw and catch a custom exception when the age of a voter is less than 18.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
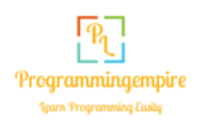