The following example code demonstrates how to Display First and Last Object from a LinkedList in Java.
Here’s an example of how to display the first and last objects from a LinkedList of Employee objects in C#.
import java.util.*;
class Employee {
private String name;
private int age;
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
public String toString() {
return name + ", " + age;
}
}
public class Main {
public static void main(String[] args) {
LinkedList<Employee> employees = new LinkedList<>();
employees.add(new Employee("John Doe", 30));
employees.add(new Employee("Jane Doe", 25));
employees.add(new Employee("Jim Smith", 35));
// Display the first object
System.out.println("First object: " + employees.getFirst());
// Display the last object
System.out.println("Last object: " + employees.getLast());
}
}
In this example, the LinkedList
class is used to store Employee
objects. The getFirst
method is used to retrieve the first object in the list, and the getLast
method is used to retrieve the last object in the list. These objects are then printed to the console.
In the similar way, you can write the above program in Java.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
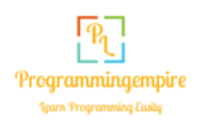