The following program demonstrates Database Connectivity in Java.
To demonstrate connectivity between Java and a database using SQL statements, you can use the JDBC (Java Database Connectivity) API. JDBC allows you to interact with relational databases using SQL queries and statements. Below is a simple Java program that shows how to connect to a MySQL database, execute SQL statements, and retrieve data.
Before running the program, make sure you have the MySQL JDBC driver (JAR file) included in your project or classpath. You can download it from the official MySQL website or use a build tool like Maven or Gradle to manage dependencies.
Here’s a basic example of connecting to a MySQL database and executing SQL statements.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class DatabaseConnectivityDemo {
public static void main(String[] args) {
// Database connection parameters
String url = "jdbc:mysql://localhost:3306/mydatabase";
String username = "your_username";
String password = "your_password";
try {
// Establish a database connection
Connection connection = DriverManager.getConnection(url, username, password);
// Create a SQL statement
Statement statement = connection.createStatement();
// Execute a SELECT query
String query = "SELECT * FROM mytable";
ResultSet resultSet = statement.executeQuery(query);
// Process the query result
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
// You can retrieve other columns as needed
System.out.println("ID: " + id + ", Name: " + name);
}
// Close resources
resultSet.close();
statement.close();
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Make sure to replace the following placeholders with your own database information:
url
: The JDBC URL of your MySQL database.username
: Your database username.password
: Your database password.
This program demonstrates the following steps:
- Import the necessary JDBC classes.
- Establish a database connection using
DriverManager.getConnection
. - Create a
Statement
object for executing SQL queries. - Execute a SELECT query using
executeQuery
. - Iterate through the query result using
ResultSet
. - Close resources (result set, statement, and connection) in a
finally
block or using try-with-resources.
Ensure that you have the MySQL database server running and the specified database and table exist with the appropriate data. Additionally, make sure the MySQL JDBC driver is correctly configured in your project or classpath.
Further Reading
Spring Framework Practice Problems and Their Solutions
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
20+ Interview Questions on Go Programming Language
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
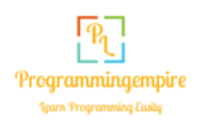