The following example shows an application using an HTTP servlet to process the form data.
- First, you need to create a servlet class that extends HttpServlet. For example.
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class MyServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Get the form data
String name = request.getParameter("name");
String email = request.getParameter("email");
// Process the form data
// TODO: Add your code here
// Set the response content type and send the response
response.setContentType("text/html");
response.getWriter().println("<h1>Form data processed successfully</h1>");
}
}
- Then, you need to map the servlet to a URL pattern in the web.xml file. For example.
<web-app>
<servlet>
<servlet-name>MyServlet</servlet-name>
<servlet-class>com.example.MyServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>MyServlet</servlet-name>
<url-pattern>/process-form</url-pattern>
</servlet-mapping>
</web-app>
- After that, create a simple HTML form that will send the data to the servlet. For example.
<html>
<body>
<form action="process-form" method="post">
Name: <input type="text" name="name"><br>
Email: <input type="text" name="email"><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
- Finally, deploy your application to a web server and access the form through a web browser. When the form is submitted, the data will be sent to the servlet and processed.
Note: This is just a basic example to get you started. You can add more fields to the form and write more complex code to process the data as per your requirements.
Another example of an HTTP Servlet for processing the form data is here.
https://www.programmingempire.com/form-data-processing-using-an-http-servlet/
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
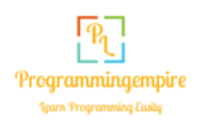