The following example demonstrates Form Data Processing using an HTTP Servlet.
Certainly, this example shows how you can develop an application using an HTTP servlet to process form data with the necessary specifications.
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class MyJuteBag extends HttpServlet {
private String groceryItems;
private String stationaryItems;
private String color = "pink";
private String material = "jute";
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Get the form data
groceryItems = request.getParameter("groceryItems");
stationaryItems = request.getParameter("stationaryItems");
// Process the form data
// TODO: Add your code here
// Set the response content type and send the response
response.setContentType("text/html");
response.getWriter().println("<h1>Form data processed successfully</h1>");
}
}
In this example, we have created a servlet class named MyJuteBag
that extends HttpServlet
. The class has two data members groceryItems
and stationaryItems
, which represents the data that we will get from the form. We have also defined two properties color
and material
with default values of “pink” and “jute” respectively.
In the doPost
method, we get the form data using the getParameter
method and store it in the groceryItems
and stationaryItems
data members. We can then process the data as needed.
In order to test this servlet, you can create a simple HTML form that sends the data to the servlet.
<html>
<body>
<form action="MyJuteBag" method="post">
Grocery Items: <input type="text" name="groceryItems"><br>
Stationary Items: <input type="text" name="stationaryItems"><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
When the form is submitted, the data will be sent to the servlet and processed. You can then customize the processing logic according to your requirements.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
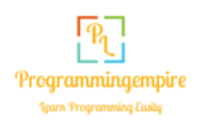