The following program demonstrates An Example of Runtime Polymorphism in Java.
When a call to a method is resolved at runtime rather than compile time, it is called Runtime Polymorphism. Furthermore, the methods are overridden methods. In other words, when a subclass redefines the methods of its superclass, with exactly the same signature, it overrides those methods. When such overridden methods are called, the method call is resolved on the basis of the type of the object. Since the objects are created at runtime, so the method call can’t be resolved until the program runs. Hence, such a type of polymorphism is called runtime polymorphism.
The following example demonstrates a base class Fruit. It defines a method eat(). Further, the Fruit class has two subclasses – Banana, and Mango. These subclasses, also define the method eat(). In other words, the classes Banana, and Mango override the method eat(). The main() method creates a reference variable of the Fruit class. Then it initializes this reference variable with the object of the Fruit class and calls the eat() method. Likewise, it initializes the reference variable of the base class with the objects of the two subclasses and calls the eat() method. The method which actually gets called depends on the type of the object.
class Fruit {
protected String name="";
protected String taste="";
protected String size="";
void eat() {
System.out.println("Fruit Name: "+name);
System.out.println("Fruit Taste: "+taste);
System.out.println("Fruit Size: "+size);
}
}
class Banana extends Fruit {
void eat() {
System.out.println("Fruit Name: Banana");
System.out.println("Fruit Taste: Sweet");
System.out.println("Fruit Size: Medium");
}
}
class Mango extends Fruit {
void eat() {
System.out.println("Fruit Name: Mango");
System.out.println("Fruit Taste: Sour");
System.out.println("Fruit Size: Large");
}
}
public class Exercise1 {
public static void main(String[] args) {
Fruit f_ob=new Fruit();
f_ob.eat();
f_ob=new Banana();
f_ob.eat();
f_ob=new Mango();
f_ob.eat();
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
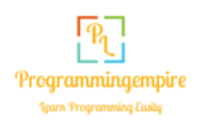