The following article demonstrates an Example of creating template objects in Django.
In Django, a template object is created by compiling a text template into an instance of the Template
class. The following code shows an example of how to create a template object in Django.
- Create a new Django app inside your project:
python manage.py startapp myapp
- Then, create a new file called “my_template.html” inside your app’s “templates” directory, and add the following HTML code.
<!DOCTYPE html>
<html>
<head>
<title>{{ page_title }}</title>
</head>
<body>
<h1>{{ page_heading }}</h1>
<p>{{ page_content }}</p>
</body>
</html>
This is a simple HTML template with three placeholders for dynamic content: page_title
, page_heading
, and page_content
.
- Open the “views.py” file inside your app’s directory, and add the following code.
from django.template import loader
from django.http import HttpResponse
def my_view(request):
context = {
'page_title': 'My Page Title',
'page_heading': 'Welcome to my page',
'page_content': 'This is some example content',
}
template = loader.get_template('my_template.html')
output = template.render(context, request)
return HttpResponse(output)
This defines a new view named “my_view” that creates a context dictionary with values for the three placeholders in the template. It then loads the template using the loader.get_template()
method, and renders the template using the template.render()
method, passing in the context dictionary and the request object.
- Finally, add a URL pattern for your new view in your project’s “urls.py” file.
from django.urls import path
from myapp.views import my_view
urlpatterns = [
path('my-template/', my_view, name='my_template'),
]
This maps the URL “/my-template/” to your new view.
Now you can run your Django development server and visit the URL “/my-template/” to see your template in action! The placeholders in the template will be replaced with the values from the context dictionary, and the resulting HTML will be sent to the user’s browser.
Further Reading
Introduction to Django Framework and its Features
Examples of Array Functions in PHP
Registration Form Using PDO in PHP
Inserting Information from Multiple CheckBox Selection in a Database Table in PHP
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
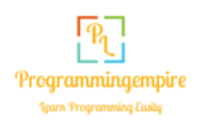