The following article describes When and how to use the ConcurrentHashMap in Java.
is a thread-safe implementation of the
ConcurrentHashMapjava.util.Map
interface in Java. It provides a way to store and retrieve key-value pairs, just like a regular HashMap
, but with the added guarantee of thread-safety.
The ConcurrentHashMap
class uses a fine-grained locking mechanism to ensure that multiple threads can access the map concurrently without any data inconsistencies. This makes it ideal for use in multi-threaded environments where multiple threads need to access the same data simultaneously.
Here is an example of how to use ConcurrentHashMap
in Java:
import java.util.concurrent.ConcurrentHashMap;
public class Main {
public static void main(String[] args) {
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
map.put("Luk", 45);
map.put("Luke", 90);
map.put("Lukette", 75);
System.out.println(map.get("Lukette"));
System.out.println(map.get("Luke"));
System.out.println(map.get("Luk"));
}
}
In conclusion, use ConcurrentHashMap
when you need a thread-safe map implementation in a multi-threaded environment. It provides fast and efficient access to the data, with the added guarantee of thread-safety.Regenerate response
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
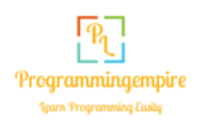