The following article describes the Difference Between Set and List in Java Collections.
In order to store collections of elements, the java.util package provides several interfaces. In fact, Set and List are two such interfaces. The following list list specifies where these interfaces differ from each other.
- Duplicate elements:
Set
does not allow duplicate elements, whileList
does allow duplicate elements. This means that when you add an element to aSet
, it checks if the element already exists in the set and only adds it if it doesn’t. On the other hand, when you add an element to aList
, it simply adds the element to the end of the list, regardless of whether the element already exists in the list or not. - Order of elements:
List
maintains the order of the elements as they were added to the list, whileSet
does not. This means that when you retrieve elements from aList
, they will be in the same order as when they were added. On the other hand, when you retrieve elements from aSet
, there is no guarantee about the order of the elements. - Index-based access:
List
provides index-based access to elements, meaning that you can retrieve an element from a specific position in the list.Set
, on the other hand, does not provide index-based access to elements.
The following example demonstrates the use of the Set interface. Since Set is an interface, you need to create an instance of the HashSet class and assign it to a reference variable of the Set interface.
import java.util.HashSet;
import java.util.Set;
public class Main {
public static void main(String[] args) {
Set<Integer> set = new HashSet<>();
set.add(77);
set.add(987);
set.add(356);
set.add(25);
for (int value : set) {
System.out.println(value);
}
}
}
Likewise, the following example code describes the usage of the List interface. Here also, you need to create the instance of a class like ArrayList class. Then again assign this instance to the reference variable of the List interface.
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> list = new ArrayList<>();
list.add(177);
list.add(245);
list.add(3555);
list.add(245);
for (int value : list) {
System.out.println(value);
}
}
}
Summary – Difference Between Set and List in Java Collections
To summarize, use Set
when you need to store a collection of elements. Furthermore, the collection needs to have all unique elements. Hence, the use of Set ensures that there are no duplicates. Whereas, you need to take advantage of the List
when you need to store a collection of elements and maintain the order of the elements as they were added.
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
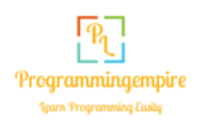