The following example code demonstrates a program Using Iterator and Enumeration on a Vector of Employee Objects in Java.
Here’s an example of using Iterator and Enumeration for a Vector of Employee objects in Java.
import java.util.*;
class Employee {
private String name;
private int age;
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
public String toString() {
return name + ", " + age;
}
}
public class Main {
public static void main(String[] args) {
Vector<Employee> employees = new Vector<>();
employees.add(new Employee("John Doe", 30));
employees.add(new Employee("Jane Doe", 25));
employees.add(new Employee("Jim Smith", 35));
// Using Iterator
Iterator<Employee> it = employees.iterator();
System.out.println("Using Iterator:");
while (it.hasNext()) {
Employee employee = it.next();
System.out.println(employee);
}
// Using Enumeration
Enumeration<Employee> en = employees.elements();
System.out.println("\nUsing Enumeration:");
while (en.hasMoreElements()) {
Employee employee = en.nextElement();
System.out.println(employee);
}
}
}
This example defines a class Employee
that has a name
and age
and creates a Vector
of Employee
objects. It then uses both Iterator
and Enumeration
to traverse the Vector
and print the name and age of each Employee
object.
Further Reading
Understanding Enterprise Java Beans
- Android
- Angular
- ASP.NET
- AWS
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Deep Learning
- Django
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- Machine Learning
- MEAN Stack
- MERN Stack
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Scratch 3.0
- Solidity
- SQL
- TypeScript
- VB.NET
- Web Designing
- XML
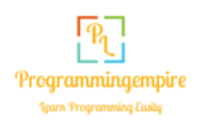