The following code shows how to perform Swapping Two Numbers Without Using Third Variable in C.
Indeed, we don’t need to use any extra variable for swapping two numbers. So, first add both variables and assign the resulting value in the first variable. After that, subtract the value of second variable from value that we obtain from the previous step and assign it into the second variable. Finally, perform the second step once again and assign the resulting value in the first variable. As a result, we get the two numbers swapped.
For instance, suppose we have two variables m and n with m=10 and n=20. Now, m=m+n=10+20=30. Further, n=m-n=30-20=10. Similarly, m=m-n=30-10=20. Hence, we get m=20 and n=10.
// Swapping Two Numbers Without Requiring Extra Variable
#include <stdio.h>
int main()
{
int x1, x2;
printf("Enter Two Numbers: ");
scanf("%d%d", &x1, &x2);
printf("\nNumbers Brfore Swapping....\n");
printf("x1=%d\nx2=%d\n", x1, x2);
//Swapping without using extra Variable
x1=x1+x2;
x2=x1-x2;
x1=x1-x2;
printf("\nNumbers After Swapping....\n");
printf("x1=%d\nx2=%d\n", x1, x2);
return 0;
}
Output
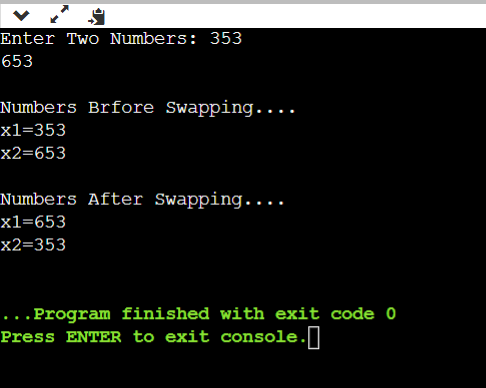
Further Reading
50+ C Programming Interview Questions and Answers
C Program to Find Factorial of a Number
- AI
- Android
- Angular
- ASP.NET
- Augmented Reality
- AWS
- Bioinformatics
- Biometrics
- Blockchain
- Bootstrap
- C
- C#
- C++
- Cloud Computing
- Competitions
- Courses
- CSS
- Cyber Security
- Data Science
- Data Structures and Algorithms
- Data Visualization
- Datafication
- Deep Learning
- DevOps
- Digital Forensic
- Digital Trust
- Digital Twins
- Django
- Docker
- Dot Net Framework
- Drones
- Elasticsearch
- ES6
- Extended Reality
- Flutter and Dart
- Full Stack Development
- Git
- Go
- HTML
- Image Processing
- IoT
- IT
- Java
- JavaScript
- Kotlin
- Latex
- Machine Learning
- MEAN Stack
- MERN Stack
- Microservices
- MongoDB
- NodeJS
- PHP
- Power Bi
- Projects
- Python
- Quantum Computing
- React
- Robotics
- Rust
- Scratch 3.0
- Shell Script
- Smart City
- Software
- Solidity
- SQL
- SQLite
- Tecgnology
- Tkinter
- TypeScript
- VB.NET
- Virtual Reality
- Web Designing
- WebAssembly
- XML