The following code shows Sorting an Array in Java Using Bubble Sort.
As can be seen in the code, array is sorted in both ascending order as well as descending order. Basically, Bubble Sort compare adjacent elements of the array in each iteration and swaps them in case the first one is bigger than the next for ascending order sorting. Further, the number of iterations are determined by number of elements in the array for the outer loop.
public class Main
{
public static void main(String[] args) {
System.out.println("Array created using command line arguments...");
int[] myarray=new int[args.length];
for(int i=0;i<args.length;i++)
{
myarray[i]=Integer.parseInt(args[i]);
}
for(int i=0;i<myarray.length;i++)
System.out.println(myarray[i]);
// Bubble Sort in Java
for(int i=0;i<myarray.length;i++)
{
for(int j=1;j<myarray.length-i;j++)
{
if(myarray[j]<myarray[j-1])
{
swap(myarray, j, j-1);
}
}
}
System.out.println();
System.out.println("Array sorted in ascending order...");
for(int i=0;i<myarray.length;i++)
System.out.println(myarray[i]);
for(int i=0;i<myarray.length;i++)
{
for(int j=1;j<myarray.length-i;j++)
{
if(myarray[j]>myarray[j-1])
{
swap(myarray, j, j-1);
}
}
}
System.out.println();
System.out.println("Array sorted in descending order...");
for(int i=0;i<myarray.length;i++)
System.out.println(myarray[i]);
}
static void swap(int[] arr, int x, int y)
{
int t=arr[x];
arr[x]=arr[y];
arr[y]=t;
}
}
Output
Array created using command line arguments…
12
4
8
98
44
563
78
16
9
94
3
85
Array sorted in ascending order…
3
4
8
9
12
16
44
78
85
94
98
563
Array sorted in descending order…
563
98
94
85
78
44
16
12
9
8
4
3
Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
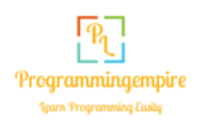