The following program demonstrates how to Search an Element in an Array in Java.
In the following code, first, we initialize the array. After that, we read the user input for the target element. The for loop iterates over all the elements of the array. It compares the target with each element of the array. When the element is found, it stores the index of the element in the variable pos, exits the for loop, and prints the index. Otherwise, it prints -1.
import java.util.*;
public class Exercise3
{
public static void main(String[] args) {
int[] myarray={3, 5, 1, 12, 9, 24, 6, 8};
int pos=-1;
System.out.println("Array Elements...");
for(int i=0;i<myarray.length;i++)
{
System.out.print(myarray[i]+"\t");
}
System.out.println("\nEnter the target element...");
int target=(new Scanner(System.in)).nextInt();
for(int i=0;i<myarray.length;i++)
{
if(target==myarray[i])
{
pos=i;
break;
}
}
System.out.println("\n"+pos);
}
}
Output

Further Reading
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
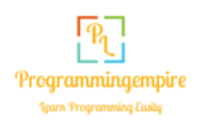