Here is the course outline for Rapid Go Programming: From Basics to Building Projects.
Embark on a fast-paced journey into the world of Go programming with our short-term course designed to equip you with essential skills and knowledge to start building projects immediately. Go, also known as Golang, is a powerful and efficient language favored for its simplicity, concurrency support, and extensive standard library. Whether you’re a beginner or an experienced developer looking to add a new language to your toolkit, this course will provide you with the foundation and hands-on experience you need to become proficient in Go programming.
Course Outline
- Introduction to Go Programming
- Overview of Go and its features
- Installing and setting up the Go environment
- Writing your first Go program: Hello, World!
- Go Language Fundamentals
- Variables, data types, and constants
- Control structures: if, else, switch
- Loops: for and range
- Functions and Packages
- Declaring and invoking functions
- Working with multiple return values
- Introduction to packages and imports
- Working with Data Structures
- Arrays, slices, and maps
- Understanding pointers
- Structs and methods
- Concurrency in Go
- Goroutines and the
go
keyword - Channels for communication and synchronization
- Handling concurrency with
sync
package
- Goroutines and the
- Error Handling
- Error types in Go
- Handling errors gracefully
- Error wrapping and custom errors
- Introduction to Testing
- Writing unit tests with the
testing
package - Running tests and analyzing coverage
- Best practices for writing testable code
- Writing unit tests with the
- Building Projects
- Designing and structuring a Go project
- Integrating third-party packages with Go modules
- Building a practical project from scratch: e.g., a simple web server, CLI tool, or API service
- Deployment and Optimization
- Compiling Go programs
- Cross-compilation for different platforms
- Optimizing Go code for performance
- Advanced Topics (Optional)
- Reflection and interfaces
- Working with JSON and other data formats
- Profiling and debugging Go applications
Duration: 4-6 weeks (depending on intensity and frequency of classes)
Target Audience
- Programmers interested in learning a fast and efficient language for building scalable and concurrent applications.
- Software engineers looking to expand their skill set with a modern programming language.
- Students and professionals seeking to enhance their career prospects in the tech industry.
Prerequisites
- Basic understanding of programming concepts (variables, control structures, functions, etc.).
- Familiarity with any programming language (e.g., Python, Java, C/C++) is helpful but not required.
Delivery Method
- Instructor-led lectures and hands-on coding sessions.
- Interactive discussions and Q&A sessions.
- Practical assignments and projects to reinforce learning.
- Online resources and documentation for further study.
At the end of the course, participants will have gained the knowledge and confidence to start building their own projects in Go, along with the ability to understand and contribute to existing Go codebases.
Mail us for queries: emailtoprogrammingempire@gmail.com
Further Reading
Features of Go Programming Language
Benefits of Go Programming Language
Variables and Data Types in Go
Go Programming Practice Exercise
Applications of Go Programming Language
20 Go Programming Practice Problems
Program Structure in Go Programming Language
Spring Framework Practice Problems and Their Solutions
How to Create and Run a Simple Program in Go?
20+ Interview Questions on Go Programming Language
From Google to the World: The Story of Go Programming Language
Why Go? Understanding the Advantages of this Emerging Language
Creating and Executing Simple Programs in Go
- Angular
- ASP.NET
- C
- C#
- C++
- CSS
- Dot Net Framework
- HTML
- IoT
- Java
- JavaScript
- Kotlin
- PHP
- Power Bi
- Python
- Scratch 3.0
- TypeScript
- VB.NET
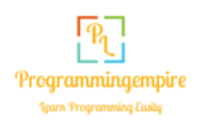